解决多个组件的状态共享,解决路由间传递复杂数据,便于维护。

目录结构
通常情况下 我们在src下建立一个store文件夹 具体如下图
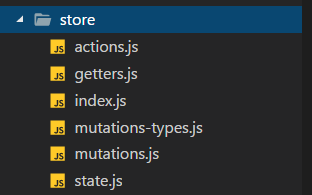
- state.js里是要用到的状态;
- getters.js是用来映射state里的状态;
- mutations.js是改变state里状态的一些方法,只能同步操作;
- mutations-types.js是统一管理mutaions方法名的一个文件(多人协作时方便管理,也可以不用);
- actions.js 不直接改变state里的状态,通过commit mutations里的方法进行改变state,并且可以分发多个mutaitons并且可以进行异步操作;
- index.js 入口文件
例子:
例子中用了export,export default 两种不同的导出语法
如果不清楚可以查阅另一篇文章 ✌ 点击进入 javascript的模块化
state.js
1 | const state = { |
getters.js (进行映射state)
1 | export const singer = state => state.singer |
mutations-types.js (统一管理mutations方法名称常量)
1 | export const SET_SINGER = 'SET_SINGER' |
mutations.js (改变state的一些方法)
1 | import * as types from './mutations-types' |
actions.js(派发多个mutations或进行异步操作)
在 store 上注册 action。处理函数总是接受 context 作为第一个参数,payload 作为第二个参数(可选)。
context包含以下属性,第二个参数可选(调用时传入的参数)
1 | { |
下面用了结构赋值 第一个参数只接收commit,有些场景也需要接收state {commit, state}
1 | import * as types from './mutations-types' |
index.js (vuex入口文件)
1 | import Vue from 'vue' |
在项目的入口文件main.js中将该 Store 实例添加到构造的 Vue 实例中
main.js
1 | import Vue from 'vue' |
利用辅助函数在组件中获取state,映射getter,提交mutation,分发action
1 | import { mapState, mapMutations, mapActions } from 'vuex' |
vuex的分割模块
以vue-element-admin的store为例 ✌ 点击传送
目录结构:
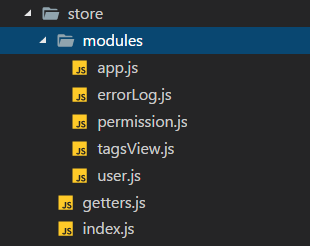
- index.js 入口文件
- getter.js state的映射
- modules模块文件夹,里面为一个个vuex模块
以errorLog模块为例
1 | const errorLog = { |
getter.js state的映射
1 | const getters = { |
index.js vuex入口文件
1 | import Vue from 'vue' |
main.js 项目入口文件
1 | import store from './store' // 加载store |
模块写法只是将state,mutations,actions写到了不同的功能模块中,并且分别加载并挂在到Vuex实例中,多人写作或者功能模块较多的项目推荐这种写法
Vuex数据持久化,结合localStorage
vuex刷新后就会消失, 很多应用场景刷新要保留数据
结合本地存储只要不手动清除,就一直存在
思路
- 最好是写一个js文件,根据业务场景写localStorage的set和get和移除或清空
- state里的数据直接获取localStorage存储的数据
- mutaions或actions方法里调用js文件中是set get 移除 清空等方法
demo
写set get方法的文件
cache.js
1 | import storage from 'good-storage' |
state.js (获取存储)
1 |
|
mutations.js
1 | import * as types from './mutations-types' |
actions.js (更新存储)
1 | import * as types from './mutations-types' |
当派发actions时会做对应的存储操作,state会拿到本地存储的数据