better-scroll是解决各种滚动场景需求的插件,由于一般移动端项目滚动的场景特别多,所以会对其进行封装复用

Vue中的better-scroll学习及使用
better-scroll 中文文档 ✌ 点击传送
首先大概了解一下该插件的滚动原理
- 父容器有一个固定高度
- 第一个子元素,高度会随内容进行变化
- 当内容高度不超过父容器不滚动,超过父容器就可以滚动
重点:better-scroll对外暴露了一个BScroll的类,我们初始化只需要neiw一个实例,第一个参数为父容器的DOM,第二个参数是一些配置。
- 初始化时会子酸父元素和子元素的高度和宽度,才能决定是否可以滚动,所以初始化时必须确保父元素与子元素已经渲染。否则会出现不可滚动的情况
- 当子元素或者父元素DOM结果发生变化(通常也就是数据发生变化)需要冲洗调用refresh()方法重新计算高度
写一个基础的scroll组件
dom结构很简单
1 | <template> |
1 | <script> |
使用上面封装的组件
引入scroll组件 并在页面注册 然后在template中使用
test1
1 |
|
将获取到的list数据传入scroll组件,scroll组件watch到data变化会重新计算高度,这是如果内容超过父容器就可以正常滑动了
test2
1 | <template> |
当一个滑动出现两个或两个以上由内容撑开高度异步获取的情况,要确保都获取到数据以后再传data,或者手动调用refresh方法 this.$refs.listWrapper.refresh()重新计算
test3 实现点击右侧字母左侧滑动到对应位置以及滑动右侧左侧滑动到对应位置
效果图
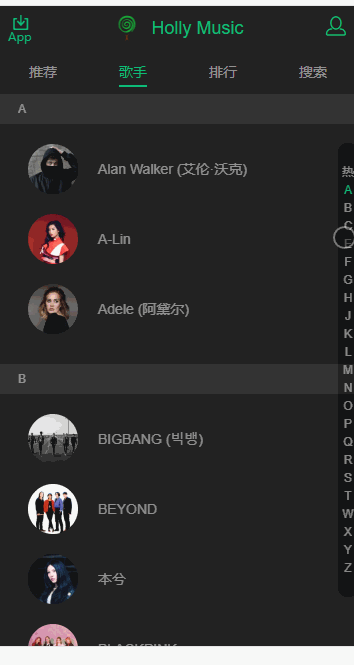
首先引入上面封装的scroll组件 并在页面注册 然后在template中使用
左侧数据与右侧数据的索引是对应好的
1 | <template> |
达到左右互相联动的效果
- 右侧点击左侧滑动到对应元素,右侧滑动,根据偏移比例左侧滑动到对应位置
- 左侧滑动到某个区间,右侧高亮
- 顶部固定title,在滑动偏移差小于title高度时,fixed有个过渡效果。